I open-sourced my Angular Template-driven Forms Solution
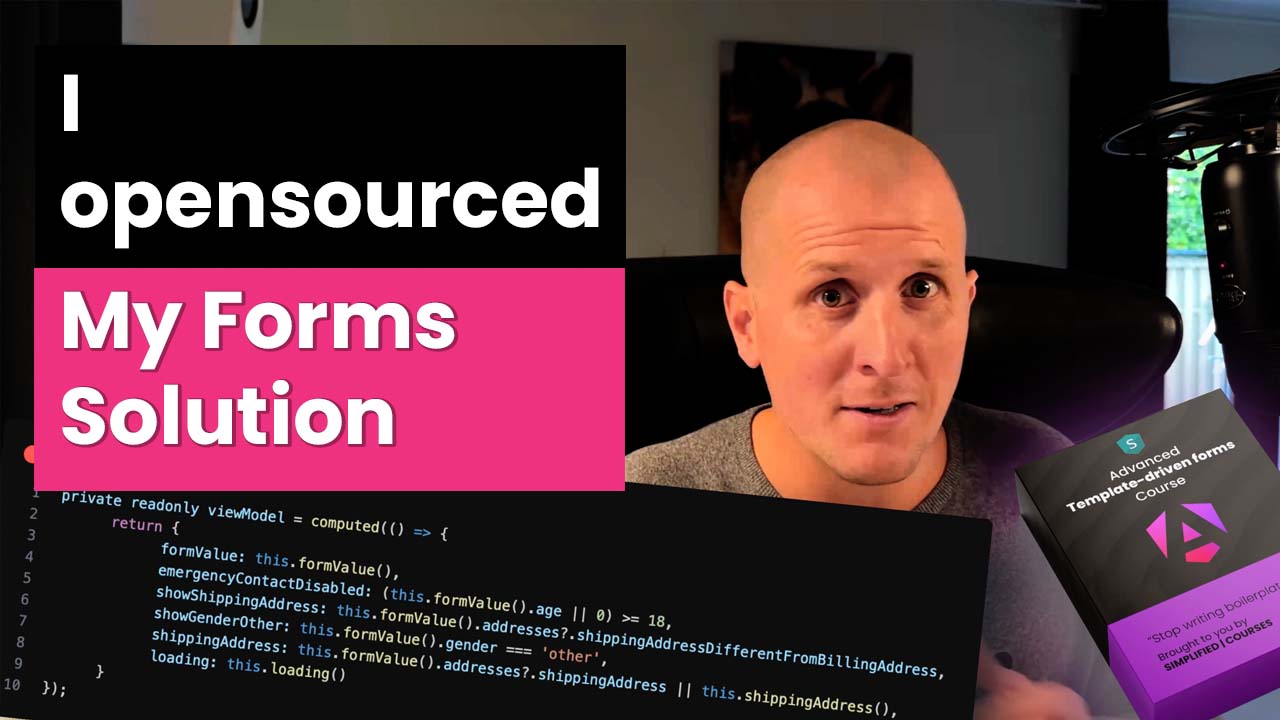
Intro
If you follow my content, you probably know that I invested a lot of time and energy in Angular Forms (specifically Template-driven Forms). I created an Advanced Template-driven Forms Course That uses an opinionated solution that focuses on:
- Boilerplate less code
- Type-safety
- Reactivity
- Declarative code
- Opinionated model validations
- Automatic validation messages translation form Vest to Angular
I care more about making a difference than sales, so I went ahead and open-sourced the entire solution. Update! now have created ngx-vest-forms Check it out here
I made a nice introduction in this article
Demo
That’s not all, I created a YouTube video where I show you how to get crazy productive with these forms. We create a completely validated form with No boilerplate at all:
This is the typescript part of that simple form…
@Component({
selector: 'app-simple-form',
standalone: true,
imports: [CommonModule, vestForms],
templateUrl: './simple-form.component.html',
styleUrls: ['./simple-form.component.css']
})
export class SimpleFormComponent {
protected readonly suite = simpleFormValidations;
protected readonly formValue = signal<SimpleFormModel>({})
}
This is the html of my form
element:
<form scVestForm
[formValue]="formValue()"
[suite]="suite"
(formValueChange)="formValue.set($event)">
...
</form>
And is the amount of code needed to create an input with automatic validation messages:
<div sc-control-wrapper>
<label>
<span>First name</span>
<input type="text" name="firstName" [ngModel]="formValue().firstName">
</label>
</div>
We will create a completely type-safe form that is:
- Unidirectional
- Has a
firstName
,lastName
,age
,emergencyContact
and 2 passwords. - Has conditional validations
- Has validations on multiple fields (Compare passwords)
- Validates on Blur
- Validates on Submit
This is the result:
I created all that in 5 minutes without any boilerplate code!
Check it out here and learn how to become extremely productive with Forms in No-time!
Note, this still uses the old solution. We have a stable api now ngx-vest-forms
Here is the Stackblitz example from the video.
Follow @TwitterDevJoin the club and get notified with new content
Get notified about our future blog articles by subscribing with the button below.
We won't send spam, unsubscribe whenever you want.
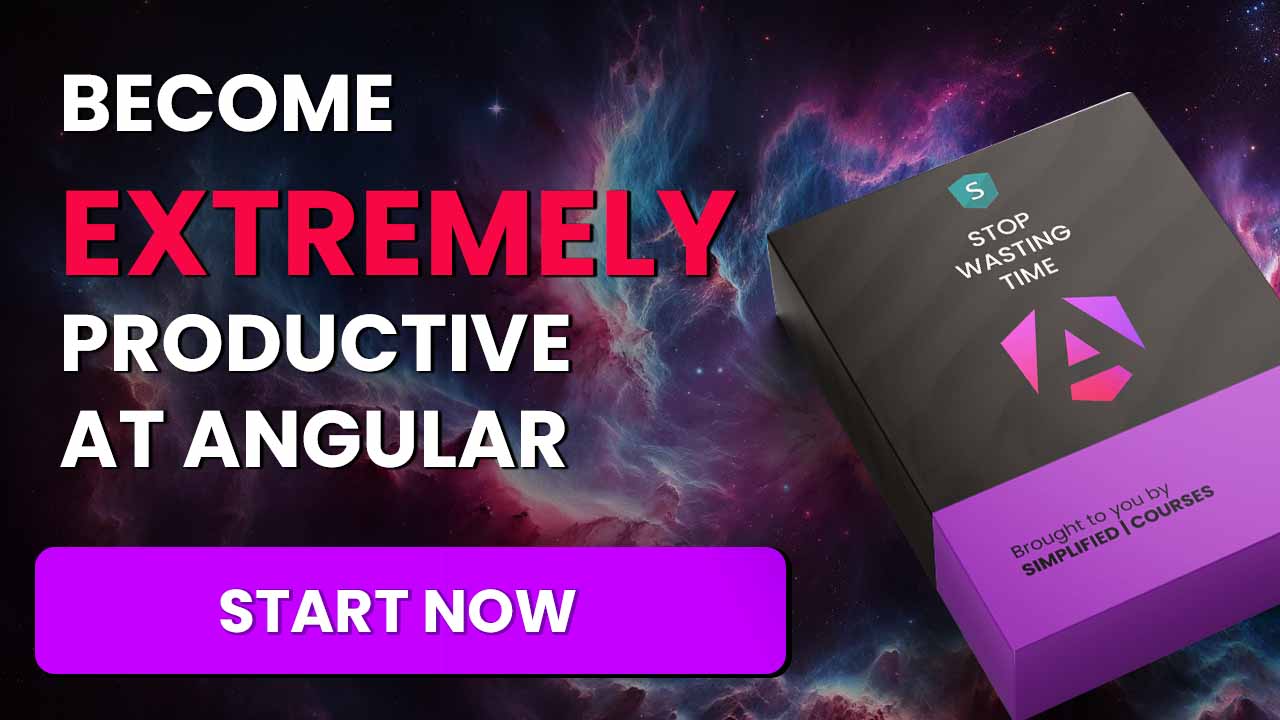
Update cookies preferences